
The datetime module contains a class also called datetime. Luckily, the hard work has already been done for us! Python includes module called datetime that handles parsing for dates and times (go figure )). Parsing dates can get hairy very quickly. Our first field is a date, however, which requires a bit more work. Converting from text to integers or floating point numbers is easy we can just call the int() or float() function.

In order to process and plot it, we need to convert each field to its appropriate type (e.g., a date, an integer, etc.). With a picture of the call stack in our heads, let's move on to parsing our workout data. A list named my_list, for example, can be copied simply by slicing the whole thing my_list. If you really need to, making copies is easy. It can lead to confusion, however, if you don't expect a function to make changes to a parameter (e.g., trying to read data from reader after calling filter_lines produces nothing since we're at the end of the file). Python copies things by reference instead of by value, which is very fast (it only needs to point the new variable at the right thing in memory). However, they point to the same file in memory, so reading from the file inside of filter_lines changes the file position of reader in the global function. It's important to remember that the reader in the global function and the reader in filter_lines are two different variables. Each time we call keep_line inside filter_lines, Python saves its place on the call stack, copies a reference to line, jumps to keep_line, and jumps back to filter_lines when it's done.
#Savefig matlab 2012 code#
When we call filter_lines with reader as a parameter, Python copies a reference to workout.csv into a new variable reader, makes a note that it should return to the global function, and jumps to the code for filter_lines. Python starts out in the "global" function whose code is just the main body of your program. Below is a diagram of our program before and after the call to filter_lines. When a return statement is found (or when the function ends), Python "pops" information off call stack to remember where it was. When Python encounters a function call, like lines = filter_lines(reader), it "pushes" information about where to come back to and then jumps to the function's code. Python tracks which functions are currently being executed with a data structure named the call stack. For that, we need to understand the call stack Before moving on to parsing the data (converting it from text to dates, integers, etc.), let's take a moment to think about how Python is actually using our filter_lines and keep_line functions.

Hooray! Our blank lines and comments are gone. Running this now produces the following output: Return len(line.strip()) > 0 and not line.startswith("#") Here's the complete code so far: import csv We can see that keep_line takes in a line and will return True when the line is not blank and not a comment. Return len(line.strip()) > 0 or not line.startswith("#"): Let's make filter_lines a bit more readable by introducing a second function called keep_line: def keep_line(line): This function will take a file reader and return a list of lines (excluding blank lines and comments). If len(line.strip()) > 0 or not line.startswith("#"): Let's write a function named filter_lines that will filter the lines in a file before the CSV reader does its thing. However, this is a common task that we might want to do again and again across programs. Unfortunately, as we can see, Python's CSV reader doesn't filter out comments or blank lines. Saving this code to a file called plot_workouts.py and running python plot_workouts.py on the command-line produces the following output: The code is fairly straightforward: import csv

To begin, let's read in the data file with Python's csv module.
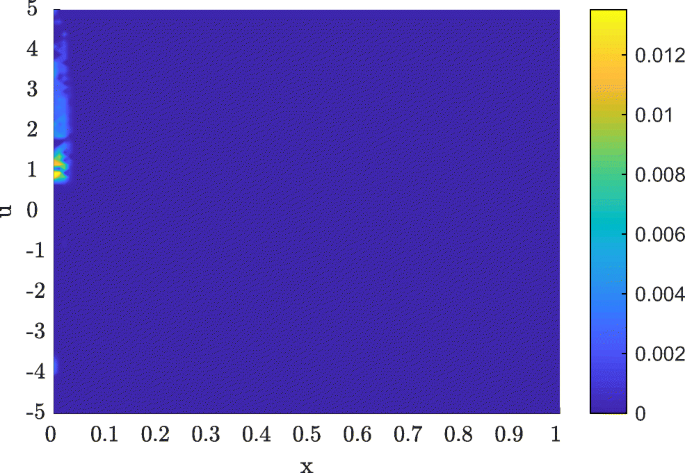
Our goal will be to read this data into Python and plot a graph with the day of the month on the x-axis and the time worked out on the y-axis. The first line (a comment) describes the fields in this file, which are (from left to right) the date of your workout, the kind of workout, how many miles you traveled, and how many minutes you spent. It's a common-separated value (CSV) file, but contains comments and blank lines. Let's say you have a text file called workout.csv that contains information about your workouts for the month of March: # date, kind of workout, distance (miles), time (min)
